
Ответ на:
комментарий
от anonymous

Ответ на:
комментарий
от anonymous

Ответ на:
комментарий
от anonymous
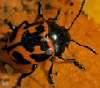
Вы не можете добавлять комментарии в эту тему. Тема перемещена в архив.
Похожие темы
- Форум Подскажите программку? (2020)
- Форум Нужна программка (2022)
- Форум Простенькая программка :( (2009)
- Форум bluetooth программка (2008)
- Форум Напишите программку! (2007)
- Форум Подскажите программку. (2005)
- Форум Подскажите программку... (2002)
- Форум Подскажите программку... (2007)
- Форум Todo программка (2014)
- Форум Подскажите, пожалуйста, программку (2011)