Выполняю упражнение 3.10 из YAHT. Нужно написать программу, которая сначала запрашивает числа(до ввода 0), после чего выводит их сумму, произведение и факториал каждого из них. В итоге должно получиться что-то вроде: Give me a number (or 0 to stop): 5 Give me a number (or 0 to stop): 8 Give me a number (or 0 to stop): 2 Give me a number (or 0 to stop): 0 The sum is 15 The product is 80 5 factorial is 120 8 factorial is 40320 2 factorial is 2 Столкнулся с проблемой перевода чисел сумм, произведений и факториалов в строки для вывода посредством putStrLn(т. е. putStrLn "The ... is " ++ number) Проблему решил с помощью функции print. Но на выходе получаеться: Give me a number (or 0 to stop): 5 Give me a number (or 0 to stop): 8 Give me a number (or 0 to stop): 2 Give me a number (or 0 to stop): 0 The sum is 15 The product is 80 5 factorial is 120 8 factorial is 40320 2 factorial is 2 [] Кто-нибудь может обьяснить, как избавиться от лишних переносов строк и правильно завершать работу рекурсивной функции(тут return [] использовалось ввиду обязательности else в конструкции if..then..else) Текст программы: module Main where import IO askForNumbers = do putStrLn "Give me a number (or 0 to stop):" number <- getLine let num = read(number) if num == 0 then return [] else do rest <- askForNumbers return (num : rest) fact 1 = 1 fact a = fact(a-1)*a factorial a = do if a /= [] then do print( head(a) ) putStrLn " factorial is " print( fact( head( a ) ) ) factorial( tail a ) else return [] main = do list <- askForNumbers putStrLn "The sum is " print( foldl (+) 0 list ) putStrLn "The product is " print( foldl (*) 1 list ) factorial list
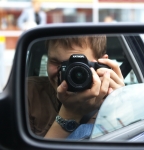
Ответ на:
комментарий
от Pi
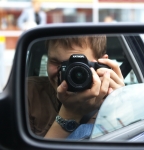
Ответ на:
комментарий
от Pi

Вы не можете добавлять комментарии в эту тему. Тема перемещена в архив.
Похожие темы
- Форум [haskell][Тормоза] (2012)
- Форум Карта фабрик на лямбдах: clang не осилил (2017)
- Форум Компоновщик ассемблера ругается (2023)
- Форум μt — C++20 библиотека модульного тестирования (2024)
- Форум Фигасе прога (2009)
- Форум init.d запускает 2 процесса при тесте. при ребуте сервис не запускается (2015)
- Статьи image viewer simply на bash (2025)
- Форум Примитивный скрипт проверки обновлений для debian (2010)
- Форум Распаковать makeself прошивку (2014)
- Форум Выясняем с помощью Emacs, какой ЯП лучше (2024)