Добрый вечер форумчане.
Появилась проблема. Такого я в своей жизни еще не встречал. Помогите пожалуйста решить, возможно это я глубоко ошибаюсь.
Код:
template<typename T>
void ArrayList<T>::realloc(int new_size)
{
// Allocating new array
T *nbuf = new T[new_size];
T *nbuf_end = nbuf + new_size;
// copying old values to new buffer
T* n_curr = nbuf;
if (itemBuf) {
T *o_curr;
for (o_curr = itemBuf; n_curr < nbuf_end && o_curr < lastItem; ++n_curr, ++o_curr)
*n_curr = *o_curr;
delete[] itemBuf;
}
itemBuf = nbuf;
itemBuf_end = nbuf_end;
lastItem = n_curr;
}
Что происходит:
При T = bool
Код
T *nbuf = new T[new_size]; // Тут все нормально
T *nbuf_end = nbuf + new_size; // Тут nbuf теряет свое значение, nbuf_end в свою очередь приобретает NULL.
При T = int
Такого не замечено. Но замеяаются другие деффекты в других методах. Если эта проблема решится, деффекты, думаю исправлю сам.
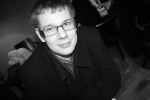
Ответ на:
комментарий
от aton

Ответ на:
комментарий
от mutable

Ответ на:
комментарий
от mutable

Ответ на:
комментарий
от mutable

Вы не можете добавлять комментарии в эту тему. Тема перемещена в архив.
Похожие темы
- Форум [g++] dynamic_cast возвращает 0x4, а не 0 — неужели баг? (2009)
- Форум Настройка потребления памяти при компиляции (2015)
- Форум boost::multi_index_container<const int> (2013)
- Форум Использование placement new (2014)
- Форум Как реализовать самозапуск чере fork+exec? (2004)
- Форум C++ жадина или я дурак? (проблема с delete) (2017)
- Форум Свой класс Any (2014)
- Форум const поля однократной инициализации в классе и оптимизация (2022)
- Форум Программа из K&R вопросы по warnings, полученные от gcc (2015)
- Форум Обмен с .so'шкой полиморфными объектами и баги компилятора? (2021)